Integrate RTL and Jest in NextJS TypeScript
09 Januari, 2023
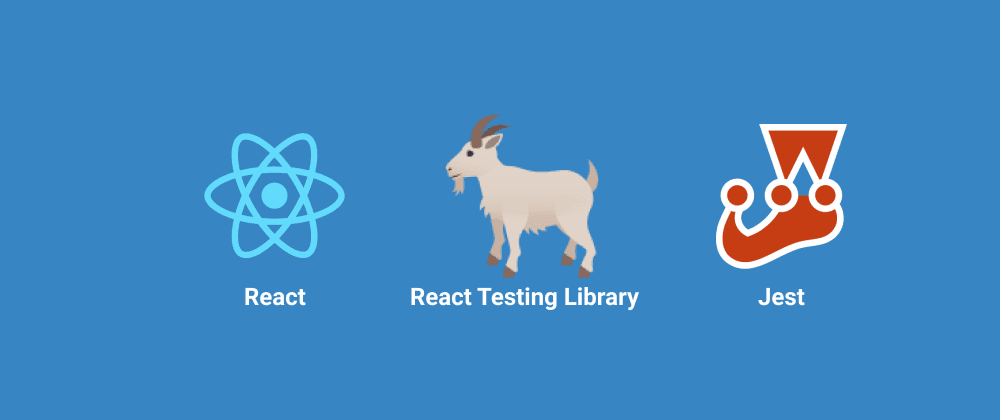
In this blog I will explain about Jest and RTL (React Testing Library) configuration with TypeScript in NextJS, but you need to know that this tutorial takes it from the official documentation. You can check it here.
If you open the link above, you will know that there are many types of unit testing. However, this time I will only discuss Jest and RTL. So let's start it
Installation & configuration
-
Create your NextJS Project first.
if you already created NextJS you can skip this step, Use your fav terminal and add this command.
Don't forget to open after it.npx create-next-app@latest my-project --typescript --eslint
-
Open the terminal and add this command.
npm install --save-dev jest jest-environment-jsdom @testing-library/react @testing-library/jest-dom
-
Create
jest.config.js
&jest.setup.js
in project's root directory.
Make sure that you already created it, and add the following code.js.config.js
const nextJest = require("next/jest"); const createJestConfig = nextJest({ // Provide the path to your Next.js app to load next.config.js and .env files in your test environment dir: "./", }); // Add any custom config to be passed to Jest /\*_ @type {import('jest').Config} _/; const customJestConfig = { setupFilesAfterEnv: ["<rootDir>/jest.setup.js"], moduleNameMapper: { "^@/components/(.*)$": "<rootDir>/components/$1", "^@/pages/(.*)$": "<rootDir>/pages/$1", }, moduleDirectories: ["node_modules", "<rootDir>/"], testEnvironment: "jest-environment-jsdom", }; // createJestConfig is exported this way to ensure that next/jest can load the Next.js config which is async module.exports = createJestConfig(customJestConfig);
js.setup.js
import "@testing-library/jest-dom/extend-expect";
-
Go to package.json file.
add this code inside the file especially in script attribute like this"scripts": { "dev": "next dev", "build": "next build", "start": "next start", "lint": "next lint", "test": "jest", "coverage": "jest --coverage" },
-
Go to tsconfig.json file.
add this code inside the file for make short paths when you call every components"paths": { "@/pages/*": ["./pages/*"], "@/components/*": ["./components/*"], }
-
Start to make unit-testing.
Before make testing, i suggest you to check on official page and learn step by step make unit testing. You can check Jest && React Testing Library For example here i would to test my dashboard pages. So inside the folder dashboard, create new folder name is__test__
and file testing index.test.jsx like thisand here is the code of
dashboard.tsx
import React from "react"; export default function Dashboard() { return ( <div> <h1>Hello World</h1> </div> ); }
here we go again...
openindex.test.tsx
file inside the__test__
folder
and the scenario is i would testing render from dashboard pages.
for exampleimport { render, screen } from "@testing-library/react"; import "@testing-library/jest-dom"; import Dashboard from "@/pages/Dashboard"; describe("Dashboard page", () => { it("Should render properly", () => { render(<Dashboard />); const header = screen.getByRole("heading"); const headerText = "Hello World"; expect(header).toHaveTextContent(headerText); }); });
-
Run testing via terminal.
add this command to your terminalnpm t
ornpm test
for testing without percentage result and usingnpm coverage
to show percentage result unit-testing.Result
Voila.. 🎉✨🎉
now you can integrate jest & react testin library in nextjs project
Source: NextJS Testing RTL & Jest